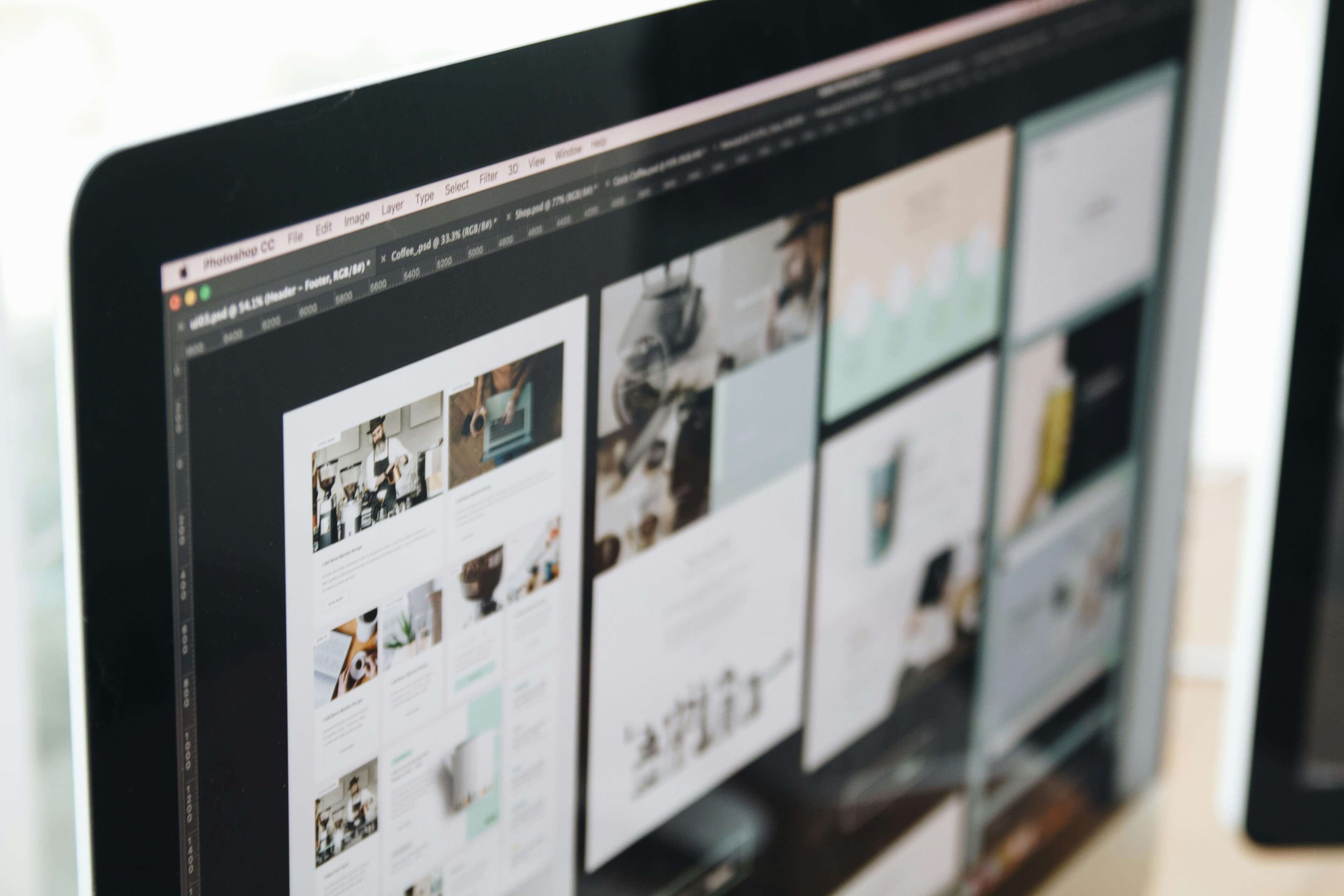
JavaScript is a language often used for asynchronous programming, which allows code to run without blocking other operations. However, managing asynchronous code can be tricky, especially when handling multiple operations that depend on each other. Promises and the async/await syntax offer a powerful solution to these challenges, making code cleaner, easier to read, and more manageable. This blog post will dive into what Promises and async/await are, how they work, and how they can improve your JavaScript code.
What Are JavaScript Promises?
A Promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation. Promises provide a way to write asynchronous code that is both readable and reliable by using three states: pending, fulfilled, or rejected. When you make a request (like fetching data from a server), the Promise starts in a pending state. It either resolves successfully (fulfilled) or encounters an error (rejected).
How to Use Promises
Promises are used with the .then()
, .catch()
, and .finally()
methods to handle the results of asynchronous operations. Here's an example of a Promise fetching data from an API:
fetch("https://api.example.com/data")
.then((response) => response.json()) // Handles success
.then((data) => console.log(data)) // Processes data
.catch((error) => console.error("Error:", error)) // Handles failure
.finally(() => console.log("Fetch attempt complete")); // Runs after success or failure
In this example, the .then()
method is used to handle successful operations, .catch()
handles errors, and .finally()
executes code regardless of the outcome. This structure makes it clear what happens at each stage of the asynchronous process.
Introduction to Async/Await
Async/await is a more recent addition to JavaScript that builds on Promises, offering a cleaner and more readable syntax. Using async
and await
allows developers to write asynchronous code that looks and behaves like synchronous code, making it much easier to follow.
An async
function always returns a Promise. Within an async function, the await
keyword pauses the function execution until the Promise settles (either fulfilled or rejected), making the code appear to run synchronously. This eliminates the need for chaining .then()
calls and handling errors in a nested manner.
How to Use Async/Await
Here’s how the same data-fetching example would look using async/await:
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data"); // Pauses here until fulfilled
const data = await response.json();
console.log(data);
} catch (error) {
console.error("Error:", error);
} finally {
console.log("Fetch attempt complete");
}
}
fetchData();
This code is functionally the same as the earlier example but is more readable and easier to maintain, especially as the complexity of asynchronous logic increases. The try...catch
block neatly handles errors, and the finally
block ensures that cleanup code runs regardless of success or failure.
Benefits of Using Promises and Async/Await
-
Improved Readability: Async/await reduces the amount of code needed for complex asynchronous operations and makes it look more like traditional synchronous code, making it easier for others (and yourself) to read and understand.
-
Error Handling: Both Promises and async/await provide structured ways to handle errors, preventing unhandled exceptions and making debugging easier.
-
Sequential and Concurrent Execution: Async/await allows you to run asynchronous code sequentially or concurrently with minimal effort, providing greater control over how and when operations are performed.
Best Practices and Considerations
When using async/await, it’s crucial to remember that all functions using await
must be inside an async
function, and each await
pauses execution until the Promise resolves. For tasks that can run independently, consider running them concurrently using Promise.all()
to avoid unnecessary delays.
Conclusion
JavaScript Promises and async/await are essential tools for handling asynchronous operations, providing a structured and readable way to manage complex workflows. Promises introduce a clear system of handling success and failure, while async/await builds on this foundation with an even more intuitive syntax. By mastering these concepts, developers can write more maintainable, efficient, and error-resistant code, making asynchronous JavaScript a smoother experience.
Having trouble fixing an issue with the functionality of your website? Contact us at www.piggybackstudios.co/work-with-us or give us a call any time at 719-828-4783 for a consultation.